Quick Reference
This section is for quick reference only, read the How the Script Works section to learn about the script and how to run it.
Usage:
python3 ae-total-render-time.py /path/to/AERenderLogsFolder/
Command Line Example
Download the script from our Github here:
...or paste the code below into a text editor, then save it as ae-total-render-time.py
ae-total-render-time.py
#!/usr/bin/env python3
# Command Line Usage: python3 ae-total-render-time.py /path/to/AERenderLogsFolder/
# Title: ae-total-render-time.py
# Description: Calculate the Total Render Time of your completed After Effects renders.
# Author: gfxhacks.com
# More Info: https://gfxhacks.com/ae-render-logs-total-render-time
import os
import sys
import datetime
class Main:
def __init__(self):
print("\nRunning...\n\n---\n")
# get folder path as passed to the command in Terminal
self.directory = sys.argv[1]
# set initial total time
self.totalTime = 0;
# set count for read log files
self.logCount = 0;
# check if folder exists, then walk
if os.path.exists(self.directory):
self.__walkDir__()
else:
sys.exit('Error: {} does not exist.\nExiting...'.format(self.directory))
def __walkDir__(self):
# walk the directory recursively
for root, subdirs, files in os.walk(self.directory):
# filter for txt files only
files = list(filter(lambda file: file.endswith('.txt'), files))
# iterate through txt files
for filename in files:
self.filename = filename
self.file_path = os.path.join(root, filename)
self.__getElapsedTime__()
else:
# when loop ends, print total time
if self.totalTime > 0:
print("\n---\n\nTotal Render Time: {}\n\n---\n".format(str(datetime.timedelta(seconds=self.totalTime))))
else:
print("No render times found here. Choose another location.\n\n---\n")
sys.exit()
def __getElapsedTime__(self):
with open (self.file_path, 'rt') as myfile:
# iterate line by line: if elapsed time exists, extract it
for line in myfile:
if "Elapsed" in line:
elapsedTime = line[line.rfind(":")+1:].strip()
self.logCount += 1
print("{}. {} reported in log file: {}".format(self.logCount, elapsedTime, self.filename))
self.__extractTime__(elapsedTime)
def __extractTime__(self, et):
# extract the elapsed time, split values by commas
if "," in et:
et = et.split(',')
for i in et:
self.__formatTime__(i.strip())
else:
self.__formatTime__(et.strip())
def __formatTime__(self, t):
# get strings
s = [s for s in t.split() if not s.isdigit()][0]
# get digits
d = [int(s) for s in t.split() if s.isdigit()][0]
# return seconds
def sc():
return d
# convert minutes to seconds
def mn():
return d*60
# convert hours to seconds
def hr():
return d*3600
f = {
"Sec": sc,
"Seconds": sc,
"Second": sc,
"Min": mn,
"Minutes": mn,
"Minute": mn,
"Hr": hr,
"Hours": hr,
"Hour": hr
}
# find whether value is Hours, Minutes, or Seconds
# convert all to seconds, then accumulate
self.totalTime += f[s]()
if __name__ == "__main__":
Main()
How the Script Works
The script can be run in Python from the Command Line (Terminal in OSX) - more about this in the Running the Script section on this page.
You'll need to specify a directory that contains AE render logs. The script will then iterate through that folder and any subdirectories, extract the Total Time Elapsed from each log file, and sum all those values up - giving you the Total Render Time.
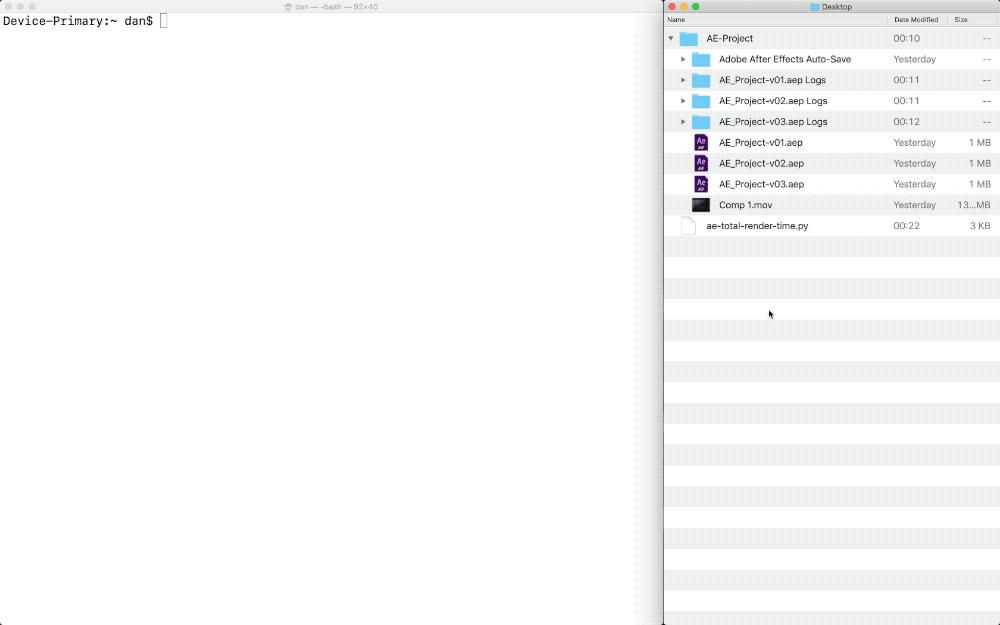
Running the Script (OSX)
The script is written in Python 3.
Note: If you are unfamiliar with Python, read the Getting Started with Python 3 section (on this page) first, to check if Python 3 is already installed on your machine and how to upgrade/install it.
Open a new Terminal window.
Now type python3
. Hit spacebar
once, then drag and drop your python script ae-total-render-time.py
to the Terminal window.
Hit spacebar
again, then drag and drop your AE render log folder. You should end up with something similar to this:
python3 ae-total-render-time.py /Users/dan/Desktop/myAEProject.aep\ Logs
Hit enter
.
The script will iterate through every log file in the specified folder, extract the Total Time Elapsed of each render job, add them together, and finally display the grand total.
About Render Logs
Every time a render is launched in After Effects, a log file is generated, detailing statistics such as when the render started, where it was output, and the Total Elapsed Time - or how long the render took.
When render completes, fails, or is cancelled, the log file is written to a folder with the project's name, in the same directory where the project file is located.
To view where the log file was written to: In the AE's render panel, simply toggle the Render Settings view of the rendered item in the queue. The full path of the log file should appear.
Note: Once a composition is added to the render queue, you can adjust the Verbosity level (level of detail) of the statistics written to the log file for that composition. Just select one of the options from the Log dropdown box. (Click on the arrow to the left of the queue item to toggle the render settings for that queue item). The default is Errors Only.
Getting Started with Python 3 (OSX)
If you are not familiar with Python follow these steps first to check if its installed on your system:
- Open the Terminal App (from the Applications folder or search for it in Spotlight)
- Type
python3 --version
orpython3 -V
. Then pressenter
on your keyboard to get the version of python installed on your Mac. If a version appears you can skip step 3. - If you get a message saying
command not found
, try the same command without the3
to check if an older version of python is installed. If it is, you will still need to upgrade to Python 3 so, either way, download the latest Python here: https://www.python.org/downloads
Note: MacOS from 10.2 (Jaguar) to 10.15 (Catalina) includes a system version of Python 2. MacOS after 10.15 (Catalina) will not include a default system Python. More info here: https://wiki.python.org/moin/BeginnersGuide/Download
Note: At the time of writing there are two Python versions available: Python 2 and Python 3. Python 3 is recommended and is required for the script to work.
Here is a digestible description of what Python is, what it does, and where it's used: https://www.pythonforbeginners.com/learn-python/what-is-python/
Resources
Log options in AE Render Settings:
Gfxhacks Gists on Github:
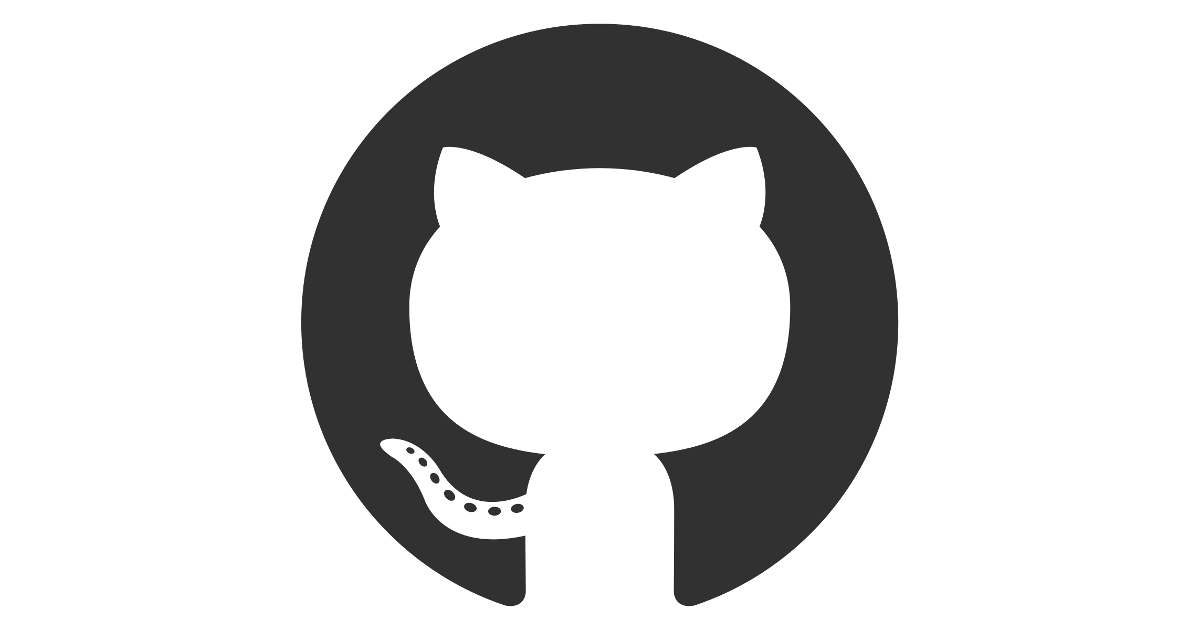
Generate project folder structures with custom templates using Python:
Reduce render times by running simultaneous renders via the command line: